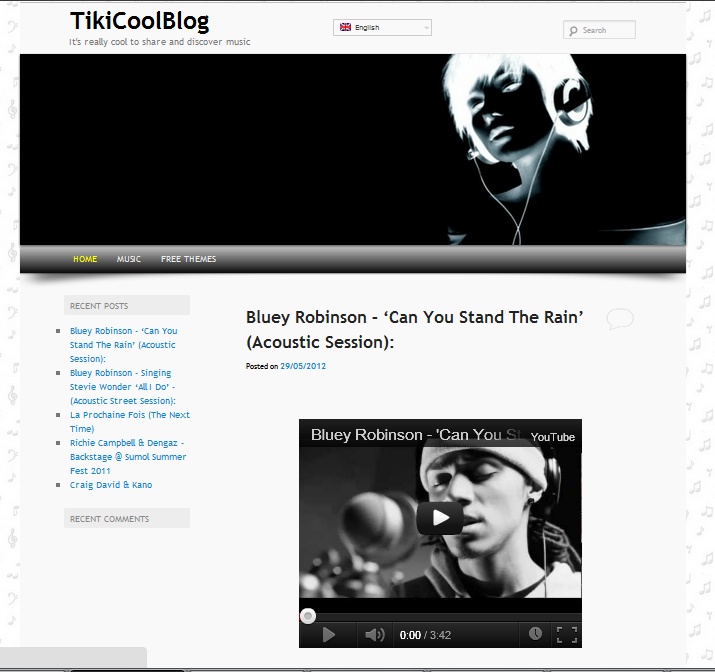
Nice blog concerning music started in.
Checkout TikiCoolBlog.
Website for Neurology Section of Serbian Medical Society.
http://www.sld.org.rs/neuroloska-sekcija/
Example for smooth scrolling button(link) is in the bottom right corner of the page.
Html(link):
<div id="top-button" class="fixed-button">
<span><a href="javascript:void(null);">top ↑</a></span>
</div>
<div id="scroll-button" class="fixed-button">
<span><a href="javascript:void(null);">bottom ↓</a></span>
</div>
Css:
.fixed-button {
background: none repeat scroll 0 0 #111111;
border: 1px dotted #444855;
color: #989eae;
bottom: 0;
right:2px;
font-size: 12px;
height: 15px;
padding: 10px;
position: fixed;
text-align: center;
width: 50px;
z-index: 800;
}
Javascript code:
$(document).ready(function()
{
if($(window).height() < $('body').height())
$('#scroll-button').show();
$(window).scroll(function(){ smoothScroller(); });
$('#top-button a').click(function(e){
$('html, body').animate({scrollTop: '0px'}, 800);
return false;
});
$('#scroll-button a').click(function(e){
$('html, body').animate({scrollTop: $('body').height() + 'px'}, 800);
return false;
});
var smoothScroller = function()
{
// get the height of #wrap
var $wrapHeight = $('body').outerHeight()
// get 1/4 of wrapHeight
var $quarterwrapHeight = ($wrapHeight)/4
// get 3/4 of wrapHeight
var $threequarterswrapHeight = 3*($wrapHeight)
// check if we're over a quarter down the page
if( $(window).scrollTop() > $quarterwrapHeight )
{
$("#top-button").show();
$("#scroll-button").hide();
}
else
{
$("#top-button").hide();
$("#scroll-button").show();
}
}
});
This is a list of WordPress plugins that I use in creating websites:
Function for text width measure in pixels:
(function($) {
$.fn.textWidth = function () {
$body = $('body');
$this = $(this);
$text = $this.text();
if($text=='') $text = $this.val();
var calc = '<div style="clear:both;display:block;visibility:hidden;"><span style="width;inherit;margin:0;font-family:' + $this.css('font-family') + ';font-size:' + $this.css('font-size') + ';font-weight:' + $this.css('font-weight') + '">' + $text + '</span></div>';
$body.append(calc);
var width = $('body').find('span:last').width();
$body.find('span:last').parent().remove();
return width;
};
})(jQuery);
Call of the function:
var $input = $(input_element_id);
var text_width = $input.textWidth();
alert(text_width);